The ChildList Field displays a list of records of a specific concept. The user can select a specific record and load the selected record to allow the user to edit the data. A button can be added to allow the creation of new records.
The ability to right-click a record is also available, which will display a list of available commands/events/wizards that can be executed on the selected record. This avoids the need to load the record and manually execute commands/events/wizards.
The records displayed in the childlist can be concept records, or custom data using a TSQL stored procedure. A refresh button can be added to enable users to manually refresh the child list data, and the data can also be set to automatically refresh at specified intervals.
A search component and/or filter button can be added on the childlist to allow the user to filter the list of records. A show grid settings button can be added to allow the user to configure the childlist grid settings.
The child list size can be configured to either the default layout or a compact layout. The number of records displayed per page can be specified, and a pager will appear to navigate through the pages. The position of the pager can also be customized.
A button to export the data can be added to the childlist to allow the user to export the childlist data as an .xlsx or .csv file.
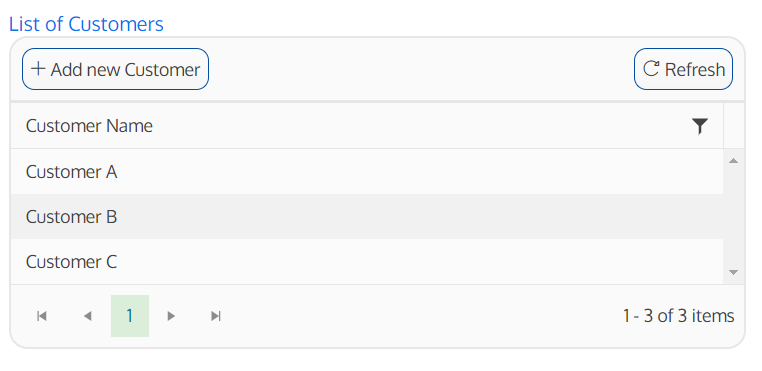
Add New Records?
Should the user be able to create new records of this Concept? If “Yes” is selected, a button to add new records of this Concept will be displayed on the childlist.
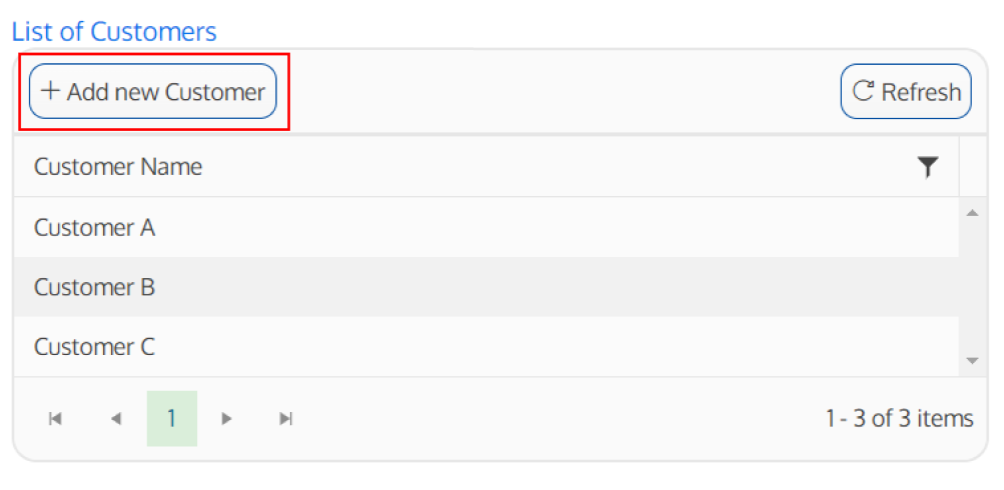
Child Concept Type
If no custom data is specified, which Concept’s records should be populated in the childlist? This will also determine the Concept type for new records if the button to add new records is enabled.
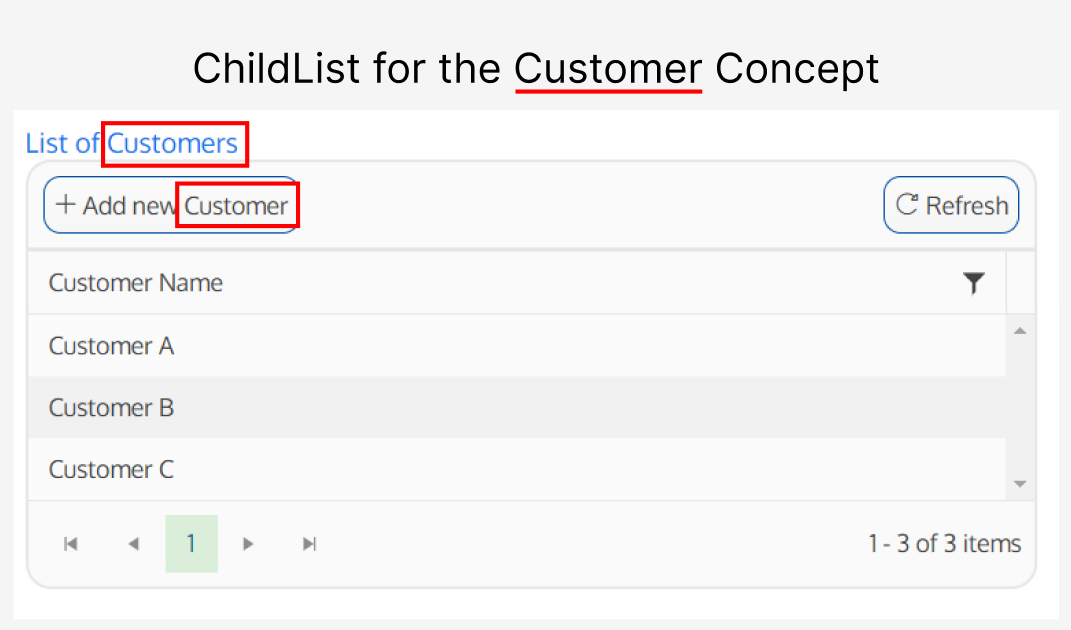
Add New Record Button Text
Only applicable when “Add New Records?” is set to “Yes”.
Overrides the text on the button that adds new records. This should be a short phrase that clearly states what will happen when the user clicks the button. If this field is left blank, the default button text will be “Add new [Concept name]”.
Add New Record Button Position
Only applicable when “Add New Records?” is set to “Yes”.
How should the button to add new records be aligned on the childlist’s top bar?
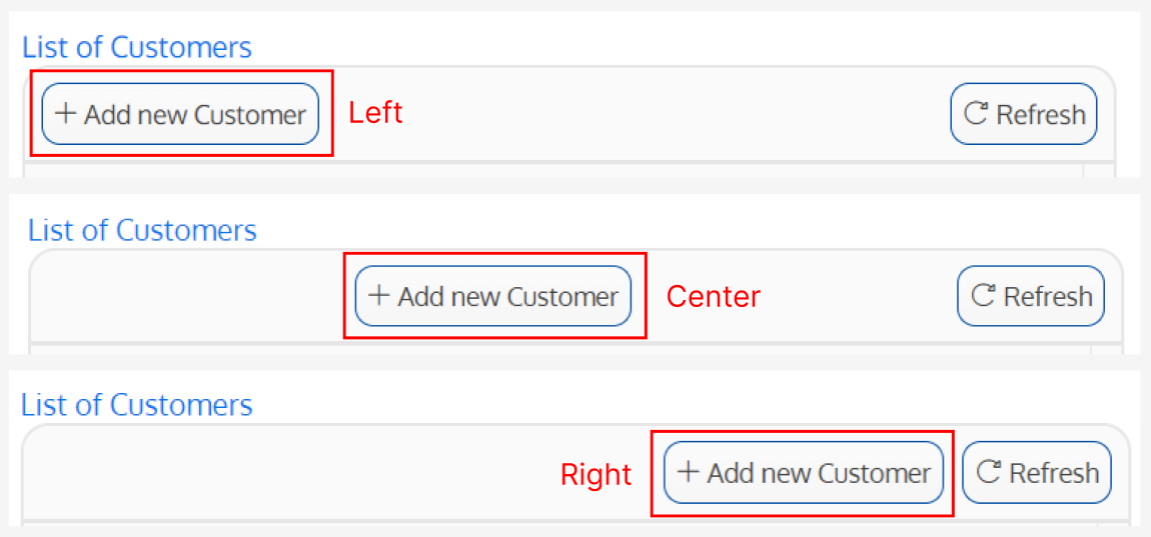
Restrict Add New Button Visibility by Condition
Only applicable when “Add New Records?” is set to “Yes”.
Specify any conditions where at least one of the conditions must be met for the button to add new records of this Concept to be displayed. These may be based on any of the Fields on this Form, the State of the record, the role(s) of the user, or some other custom action.
If Conditions are specified, a user will be able to see the button if any of the Conditions are met. To specify multiple Conditions that must be met in order enable visibility, create a Condition that encompasses all necessary criteria and add it to this Field.
The list of possible Conditions is maintained at the Form level under the Security Tab.
Do You Need to Customize the Grid Columns?
Determines if you’re getting your data from a target or not. By default, the childlist format consists of a single column with the Concept’s Instance Name. If custom columns and/or data should be displayed instead, they can be configured here.
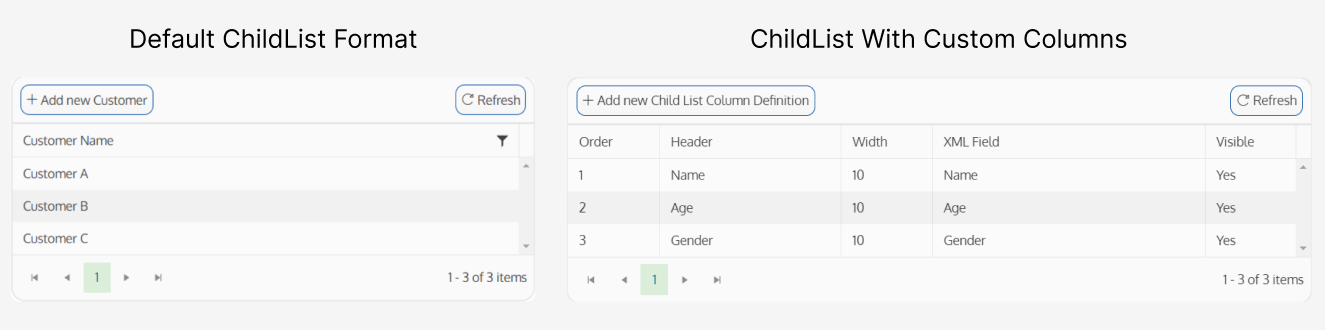
Where Do We Get the Data for This Control
Only applicable when “Do You Need to Customize the Grid Columns?” is set to “Yes”.
Contains the target information of the target that will be called to get the childlist data.
ChildList Columns
Only applicable when “Do You Need to Customize the Grid Columns?” is set to “Yes”.
The childlist Columns field enables the specification of various columns to be included in the childlist.
When data for a childlist is being returned from a target, only the bare minimum amount of data is returned. For example: if a target retrieves data from TSQL and returns five columns, only those columns with matching definitions will be retained. The others will be discarded.
The only columns that will always be returned (assuming the target populates and returns them) are Hierarchy, InstanceGUID, and InstanceName.
Each column must specify the following fields:
- Column Sequence: The order of columns from left to right, 1 being the first or furthest column to the left.
- XML Field Name: The name of the XML element that will store the column data. This field must not contain spaces.
- Column Subtype: The type of data displayed in the column. Specifying an accurate data type will provide accurate filtering options for the column. Most fields, such as strings, will use the subtype Data, but field types that require specific formatting or filter options such as DateTime, HTML, or Numeric fields will have to be specified to enable filtering controls specific to their data type. If the Column Subtype is set to DateTime and the date value in the column includes a time portion, the datetime is assumed to be UTC time and is converted to the user’s local time. If no time portion is specified, no conversion is done.
- Is Visible: Determines if the column should be visible to the user viewing the childlist. This is useful when non-human readable data needs to be included in the childlist, but it doesn’t make sense to display it.
- Column Header: This is the name that will be used for the column, this should be a human readable version of the XML Field Name value.
- Column Width: Specifies the width of the column as a percentage.
The following fields are optional:
- Horizontal Alignment: Determines how the title and text should be aligned horizontally within the column – whether it should be left aligned, right aligned, or centralized.

- Cell Style Name: The name of column returned by the TSQL that will contain the raw CSS to be applied to the cells in this column.
- Cell Class Name: The name of the column returned by the TSQL that will contain the class name to be applied to the cells in this column.
- Data Format: Only applicable to columns whose data type can be formatted, such as dates. This allows the user to determine what information should be displayed and how it should be formatted for these data types.
Add Search Component
This is an advanced feature and requires custom TSQL. Search Components are only applicable when Custom Data is selected. If a search component is defined, a TSQL procedure must also be selected.
The search component will appear at the top of the Field, and the user will be able to use it to filter the data.
Show Childlist on Initial Load When Using Search
When using a search component, the childlist will not be displayed by default until the Search button is selected. To display the childlist by default, set this field to “Yes” and ensure the target retrieving the data can handle cases with no search parameters.
Show Refresh Button
Determines if the Refresh button is visible on the childlist. This button allows the user to refresh the childlist data whenever they wish. It’s recommended to show this button if the childlist data is frequently updated.
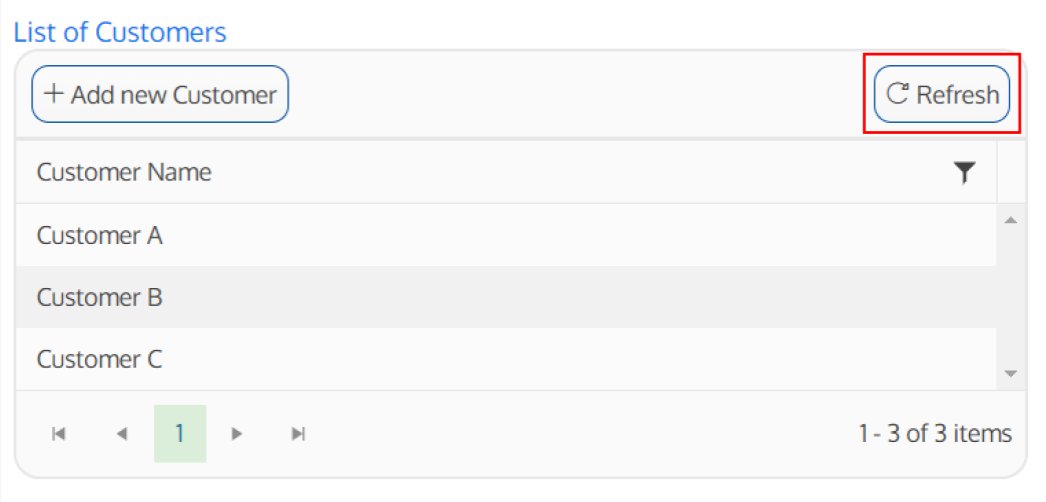
Show Grid Settings Button
Determines if the Settings button is visible on the childlist. This button allows the user to configure the grid layout of the childlist including the option to use a filter, the number of rows per page, and hiding columns.
Note: This button will allow the user to override the configurations set for “Allow Filtering” and “Page Size”.
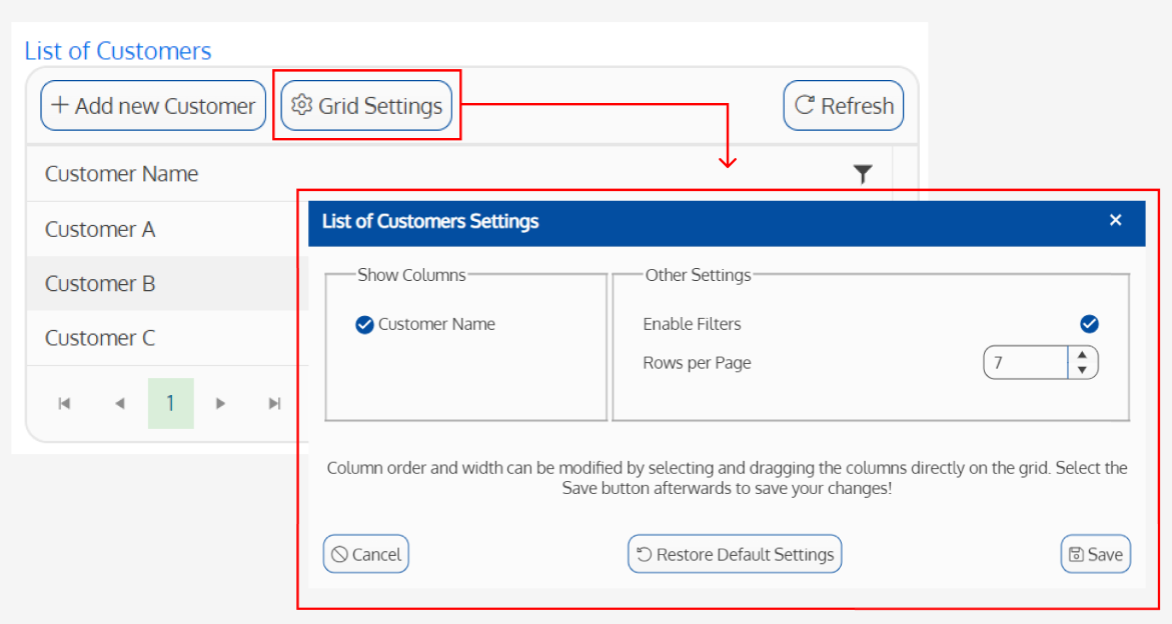
Show Export Data Button
Determines if the Export button is visible on the childlist. This button allows the user to export the data in the childlist as an .xlsx or .csv file.
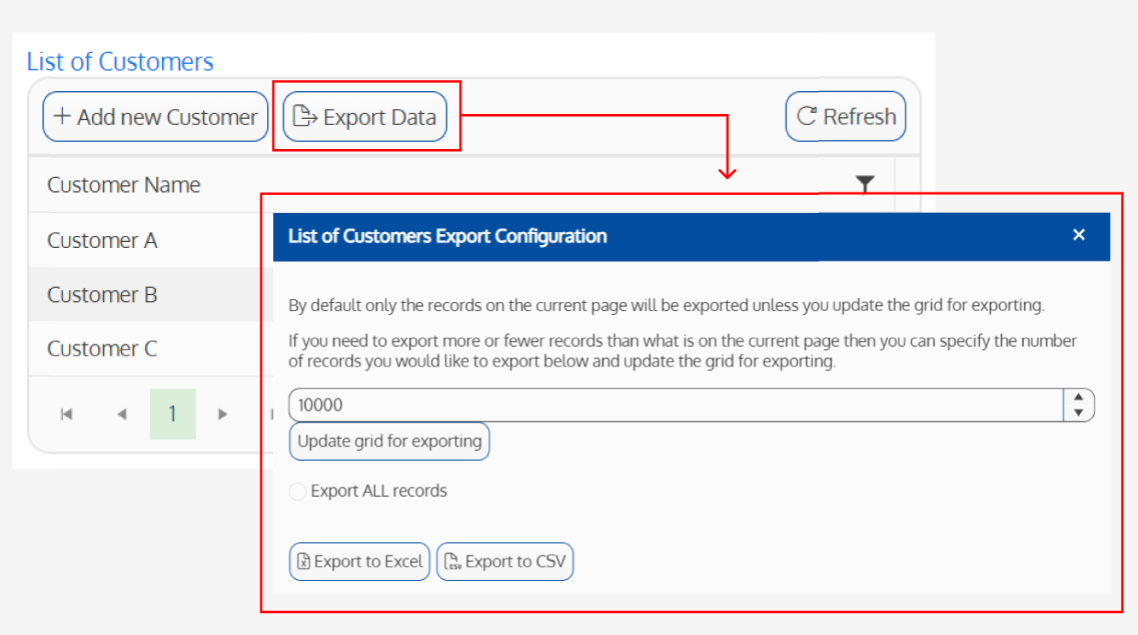
Allow Filtering
Should the user be able to filter the childlist? If “Yes” is selected, the user will have access to a filter button, allowing them to specify criteria to filter the childlist.
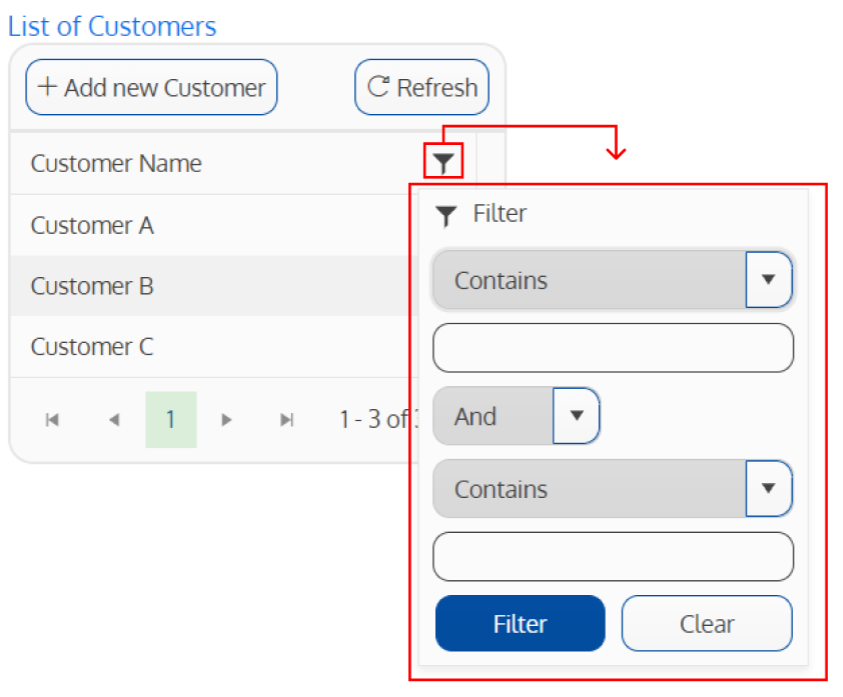
ChildList Size
Should the records be displayed in the default format or in a compact format? The default format contains more padding, while the compact format contains less padding and takes up less space.
It is recommended to use the compact format when displaying a large volume of records to allow more records to fit in the space.
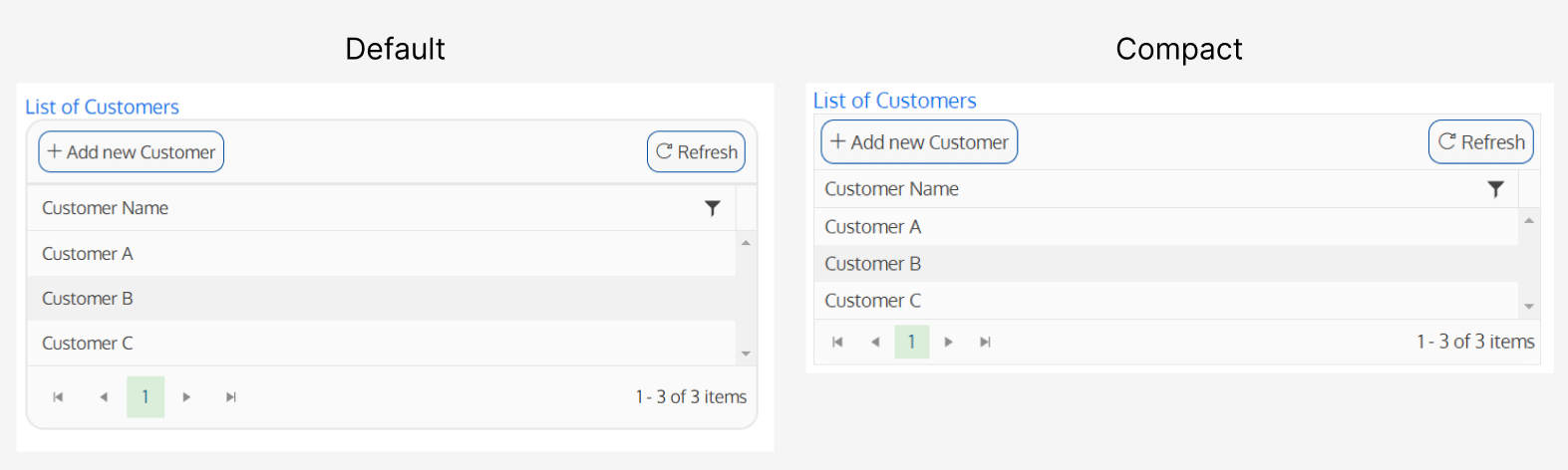
Pager Position
Determines the position of the pager on the childlist. The pager allows the user to navigate between pages.
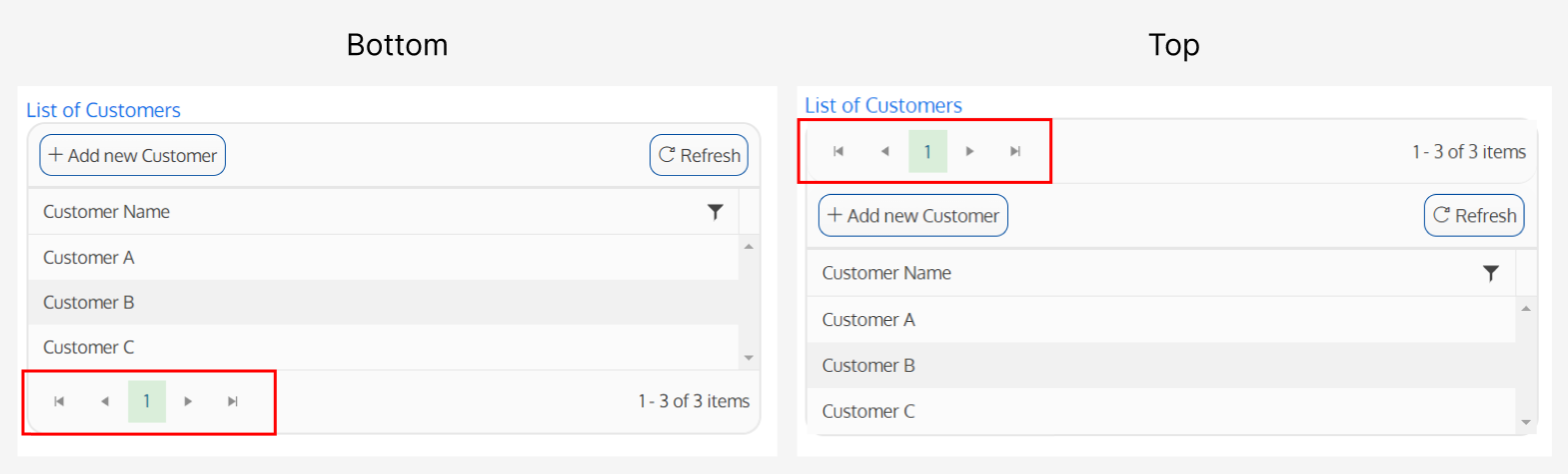
Default Page Size
How many records should be displayed on each page of the childlist? If there are more records than the Default Page Size, they will be paginated to allow navigation between pages.
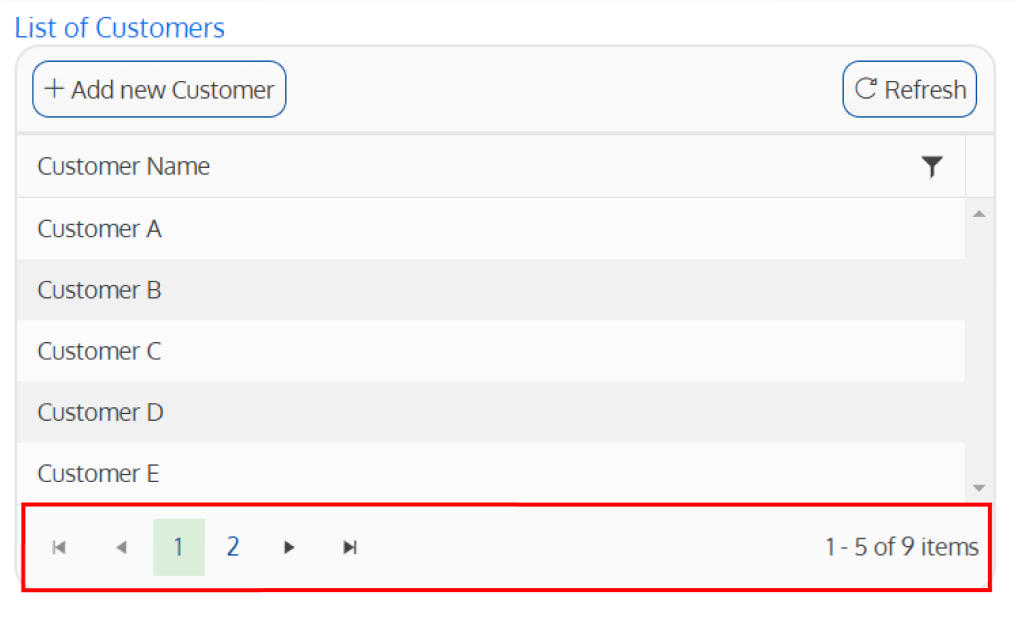
Text to Display for Empty Childlist
What text should be displayed on the childlist when the childlist is empty? If no text is entered in the Field, the text “No records available.” will be displayed when the childlist is empty.
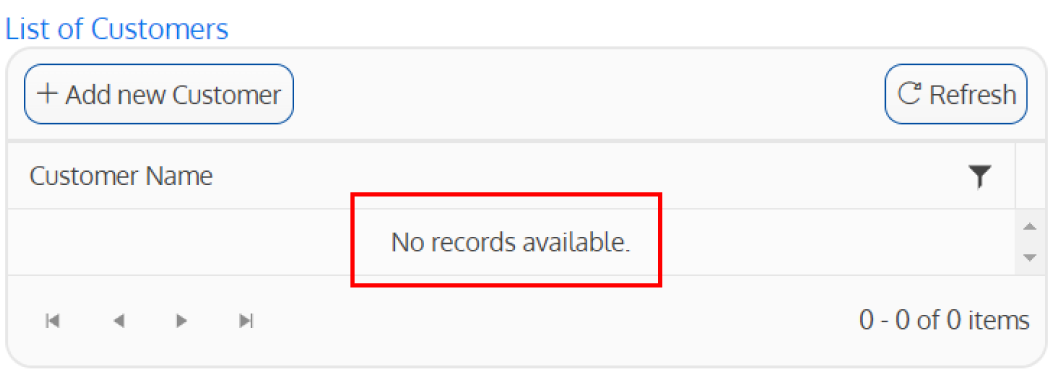
Enable Data Auto Refreshing
Determines if the childlist data will refresh automatically after a specified period of time. It’s recommended to enable this if the data is frequently updated.
Every How Many Minutes Should the Data Refresh?
Only applicable when “Enable Data Auto Refreshing” is set to “Yes”.
Specifies the interval in minutes for automatically refreshing the childlist data.
Restrict Row Click by Condition
Specify any conditions where at least one of the conditions must be met for the user to be able to click on and view records in the childlist. These may be based on any of the Fields on this Form, the State of the record, the role(s) of the user, or some other custom action.
If Conditions are specified, a user will be able to click on and view records in the childlist if any of the Conditions are met. To specify multiple Conditions that must be met in order enable this action, create a Condition that encompasses all necessary criteria and add it to this Field.
The list of possible Conditions is maintained at the Form level under the Security Tab.
Suppress Commands/Events/Wizards
When right-clicking on a row in the childlist, an action menu opens, allowing the user to execute various actions.
The Commands/Events/Wizards entered in this Field will be suppressed from this menu. Separate items with a comma (example: Delete,Open In Window).
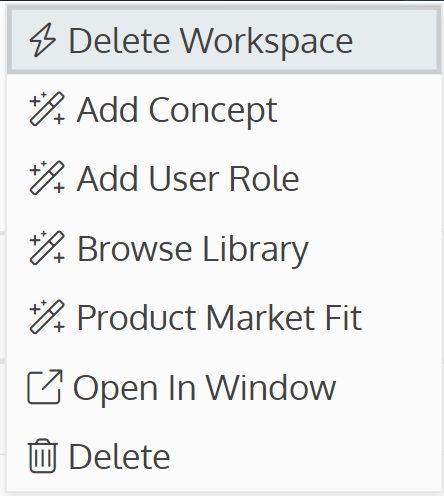
Open Instances in Popup Window by Default
When clicking on a record in the childlist, should the record open in a pop up window? If “Yes” is selected, the record will open in a pop up window. If “No” is selected, the record will open on a new page.
Custom Instance Window Title
Determines if a custom title will be applied to the instance window if the childlist row record is opened in a popup window.
If set to “No”, the window title will be the instance name.
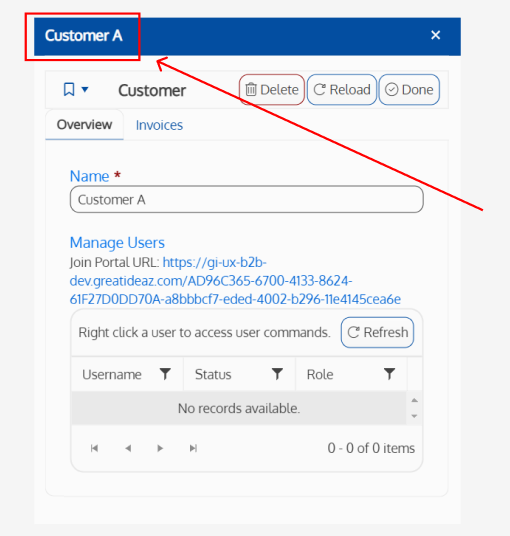
Instance Window Title
Only applicable when “Custom Instance Window Title” is set to “Yes”.
Contains the title that will be displayed when the childlist row record is opened in a popup window.